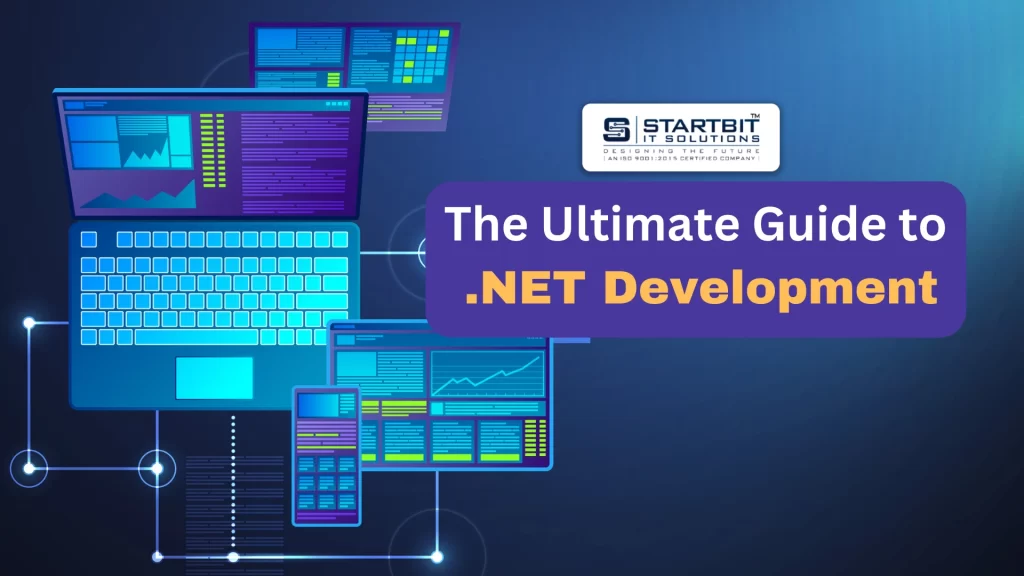
.NET is a free, open-source, cross-platform framework developed by Microsoft. It provides a runtime environment and a set of libraries for building and running various types of applications, including web, desktop, mobile, gaming, and IoT (Internet of Things) applications. The framework supports multiple programming languages, such as C#, F#, and Visual Basic, allowing developers to choose the language that best suits their needs.
.NET is designed to be versatile and interoperable, enabling developers to build applications that can run on different operating systems, including Windows, macOS, and Linux. The framework includes a Common Language Runtime (CLR), which manages the execution of code, and a comprehensive class library that provides a wide range of pre-built functionality.
What is a .NET implementation?
A .NET implementation refers to the runtime and libraries that enable the execution of applications developed using the .NET framework. .NET implementations provide a common runtime environment for diverse programming languages, facilitating cross-language interoperability. Examples of .NET implementations include the official Microsoft .NET Framework, .NET Core, and the open-source .NET 5 and later versions.
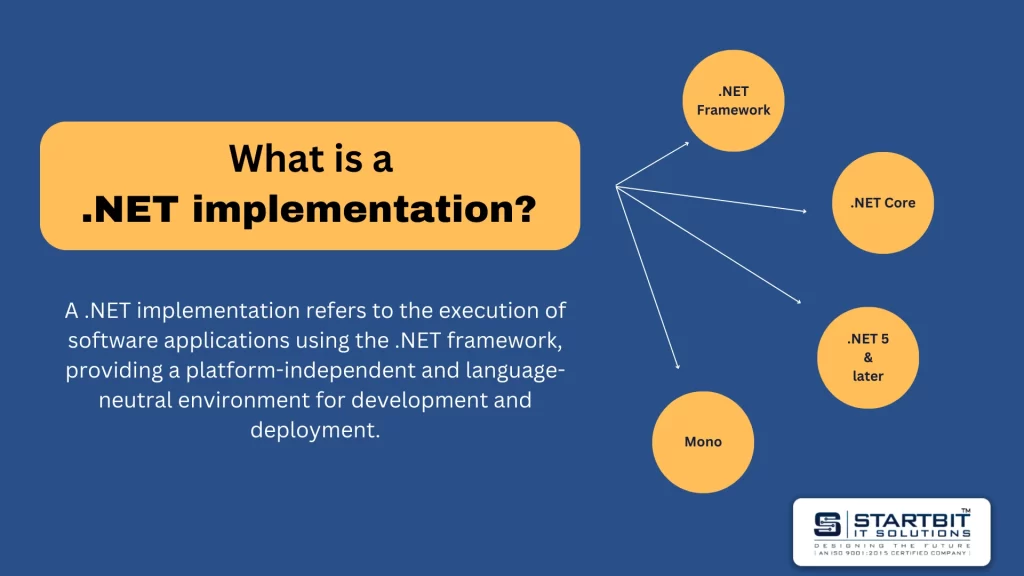
There are multiple implementations of .NET, each serving different purposes:
- .NET Framework: This is the original implementation, primarily designed for Windows. It includes a runtime (Common Language Runtime – CLR) and a set of libraries for building Windows applications.
- .NET Core: Introduced to address cross-platform development, .NET Core is a modular and lightweight implementation of the .NET framework. It supports development for Windows, macOS, and Linux and is suitable for building a wide range of applications, including web applications and microservices.
- .NET 5 and later (now .NET 6, .NET 7, etc.): With the release of .NET 5, Microsoft merged .NET Framework and .NET Core into a single platform. Subsequent versions, like .NET 6, .NET 7, etc., continue the evolution of the unified platform, providing improved performance, language features, and broader platform support.
- Mono: An open-source implementation of the .NET framework that supports multiple platforms, including Linux, macOS, and Windows. It is often used for developing cross-platform applications and games.
Why choose .NET?
Choosing .NET for development offers several advantages, making it a popular choice for various types of applications. Here are some reasons why you might consider using .NET:
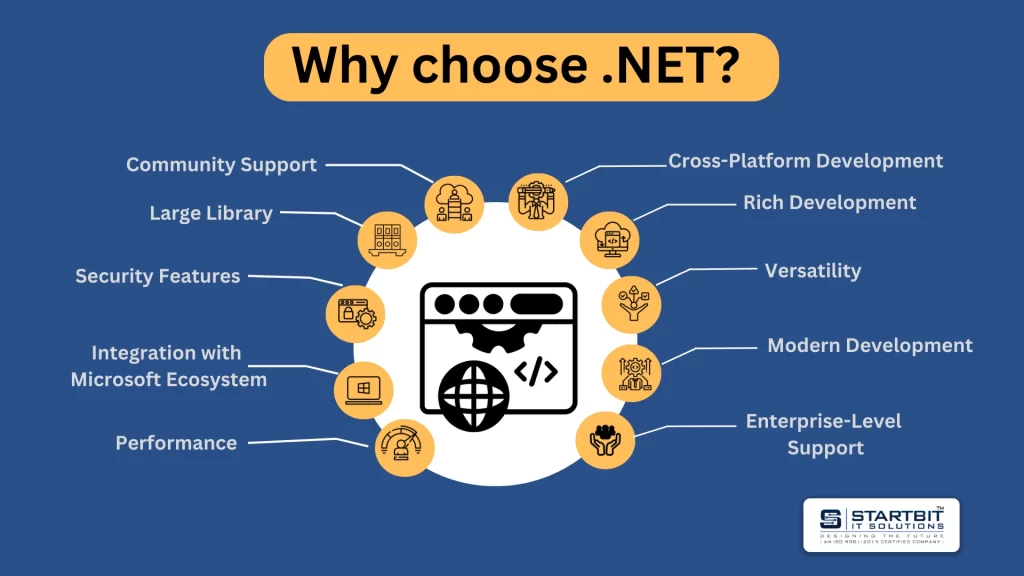
- Versatility: .NET is a versatile framework that supports multiple programming languages, including C#, F#, and VB.NET. This flexibility allows developers to choose the language that best fits their project requirements and expertise.
- Cross-Platform Development: With the introduction of .NET Core (now known as .NET 5 and later), .NET has become a cross-platform framework. You can build applications that run on Windows, Linux, and macOS, providing flexibility in deployment.
- Rich Development Environment: Visual Studio, the primary integrated development environment (IDE) for .NET, is a robust and feature-rich tool. It offers powerful debugging, profiling, and testing capabilities, enhancing the development process.
- Strong Community Support: .NET has a large and active community of developers. This community contributes to a wealth of resources, including documentation, forums, and third-party libraries, which can be beneficial when facing challenges or seeking best practices.
- Security Features: .NET provides built-in security features, such as code access security, role-based security, and encryption libraries. This helps developers implement security measures more easily and effectively.
- Performance: .NET applications are known for their good performance. The Just-In-Time (JIT) compilation and runtime optimization contribute to efficient code execution.
- Integration with Microsoft Ecosystem: If your project involves integration with other Microsoft technologies, such as Azure cloud services, SQL Server, or SharePoint, .NET provides seamless integration and compatibility.
- Large Standard Library: .NET comes with a comprehensive standard library that includes a wide range of pre-built classes and functions. This library simplifies development tasks and accelerates the coding process.
- Enterprise-Level Support: .NET is often chosen for enterprise-level applications due to its scalability, stability, and long-term support from Microsoft. This makes it a reliable choice for building large-scale, mission-critical applications.
- Modern Development Practices: .NET embraces modern development practices, such as microservices architecture, containerization (using Docker), and continuous integration/continuous deployment (CI/CD) workflows, making it well-suited for modern application development.
What are the components of .NET architecture?
.NET architecture is a versatile framework developed by Microsoft for building and running applications. It consists of several key components that work together to provide a comprehensive development and runtime environment. The main components of .NET architecture include:
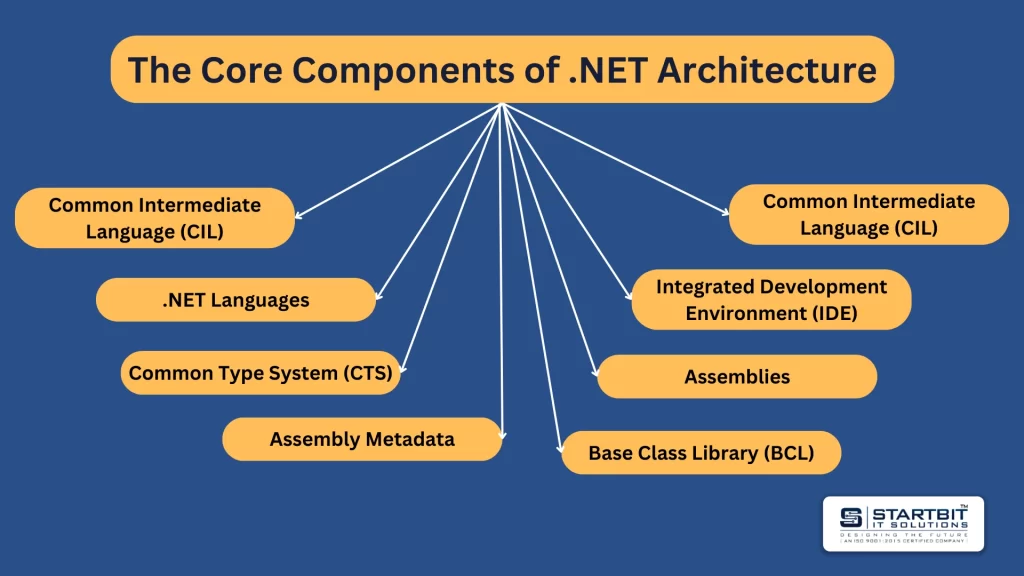
- Common Language Runtime (CLR): The CLR is a crucial component that manages the execution of .NET applications. It provides services such as memory management, security, code verification, and exception handling. The CLR ensures that .NET applications can run on any system that has the appropriate version of the .NET runtime installed.
- Base Class Library (BCL): The .NET Class Library is a collection of pre-built, reusable code that developers can use to perform common tasks. It provides a wide range of functionality, such as file I/O, networking, data access, and more. This library simplifies development by eliminating the need for developers to write code from scratch for routine tasks.
- Common Type System (CTS): CTS defines the data types and operations supported by the CLR. It ensures that types are compatible across different .NET languages, enabling seamless interoperability between languages like C#, VB.NET, and others.
- Common Intermediate Language (CIL): Also known as MSIL (Microsoft Intermediate Language) or bytecode, CIL is an intermediate language that is generated by the .NET compiler. It serves as the common language that the CLR understands, allowing .NET applications to be language-agnostic.
- Assemblies: Assemblies are the building blocks of .NET applications and can be either executables (with a .exe extension) or libraries (with a .dll extension). Assemblies contain compiled code, metadata, and resources required for the application. They support versioning, deployment, and security features.
- Assembly Metadata: Metadata within assemblies contains information about the types, methods, and other components in the assembly. It is used by the CLR for various purposes, including type checking, reflection, and runtime optimization.
- .NET Languages: .NET supports multiple programming languages, such as C#, VB.NET, F#, and more. Each of these languages can be used to build applications that target the .NET framework. The Common Language Specification (CLS) defines a set of rules that languages must follow to ensure compatibility and interoperability.
- Integrated Development Environment (IDE): Visual Studio is the primary IDE for .NET development. It provides a rich set of tools for coding, debugging, and testing .NET applications. Developers can also use other editors or IDEs that support .NET development.
What are .NET programming languages?
.NET is a cross-platform framework developed by Microsoft that supports multiple programming languages. Some of the primary languages used in .NET development include:

- C#: C# (pronounced “C-sharp”) is a versatile, object-oriented programming language designed by Microsoft. It is the most commonly used language in .NET development and is known for its simplicity, type safety, and modern features.
- Visual Basic .NET (VB.NET): VB.NET is an evolution of the classic Visual Basic language, adapted to work with the .NET framework. It provides an easier transition for developers familiar with Visual Basic and offers modern language features.
- F#: F# is a functional-first programming language in the .NET ecosystem. It supports functional, imperative, and object-oriented programming paradigms, making it suitable for various types of applications.
- Managed C++: C++ can also be used with .NET through Managed C++. It allows developers to use the features of both C++ and the .NET framework in their applications.
What is .NET runtime?
.NET runtime, also known as Common Language Runtime (CLR), is a component of the Microsoft .NET framework. It is responsible for managing and executing .NET applications, providing a runtime environment for them to run efficiently. The .NET runtime performs several key functions:
- Just-In-Time Compilation (JIT): When a .NET application is executed, the source code is not directly executed by the computer’s hardware. Instead, it is compiled into an intermediate language called Common Intermediate Language (CIL). The CLR then uses the Just-In-Time Compiler to convert the CIL into native machine code that can be executed by the underlying hardware.
- Memory Management: CLR manages the memory used by .NET applications, handling tasks such as memory allocation and garbage collection. Garbage collection helps automatically reclaim memory occupied by objects that are no longer in use, reducing memory leaks and enhancing application stability.
- Exception Handling: CLR provides a robust exception handling mechanism that allows developers to handle errors and exceptions in a structured and predictable manner, improving the reliability of .NET applications.
- Security: .NET runtime implements various security features, including code access security and role-based security, to help protect applications from malicious code and unauthorized access.
- Type Verification and Type Safety: CLR ensures type safety by verifying the types used in a .NET application, preventing common programming errors related to data types and enhancing the reliability and security of the code.
- Assembly Loading and Execution: CLR is responsible for loading and executing assemblies, which are the fundamental units of deployment for .NET applications. Assemblies contain compiled code, metadata, and resources required for the application.
- Interoperability: CLR supports interoperability with components written in other languages, enabling .NET applications to use libraries and components developed in languages like C++, COM, or other .NET languages.
What are .NET application model frameworks?
The .NET application model frameworks refer to various frameworks and technologies within the Microsoft .NET ecosystem that developers can use to build diverse types of applications. These frameworks provide a foundation and set of tools for creating applications with specific characteristics or for targeting particular platforms. Some prominent .NET application model frameworks include:
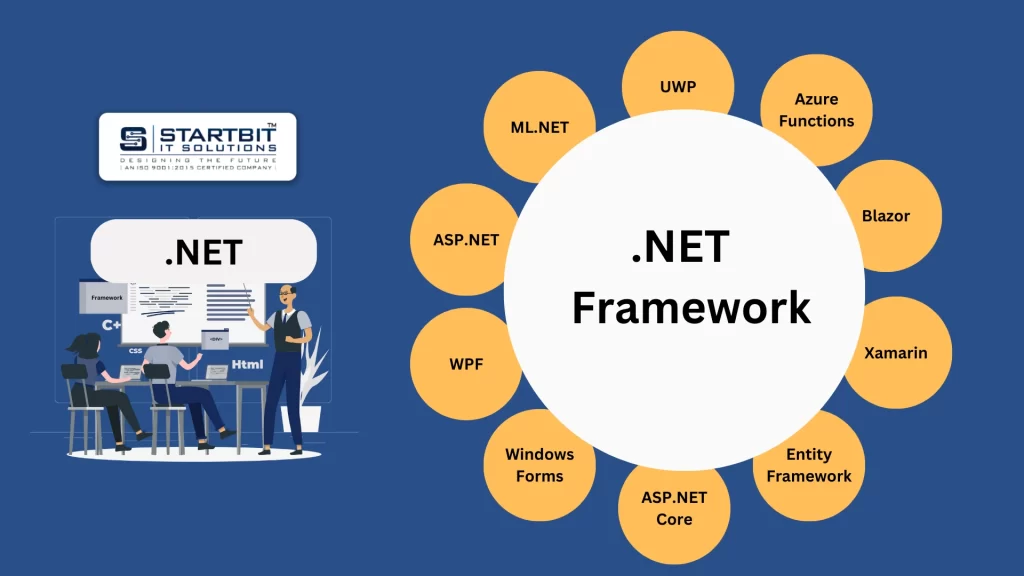
- ASP.NET:
- ASP.NET Web Forms: A web application framework for building dynamic, data-driven web applications using a drag-and-drop, event-driven model.
- ASP.NET MVC (Model-View-Controller): A web development framework that separates an application into three interconnected components (Model, View, Controller), providing better code organization and testability.
- Windows Presentation Foundation (WPF): A framework for building Windows desktop applications with rich user interfaces, supporting data binding, styling, and multimedia integration.
- Windows Forms: A framework for building traditional Windows desktop applications with a graphical user interface (GUI), using forms for designing application layouts.
- ASP.NET Core: A cross-platform, high-performance framework for building modern, cloud-based, and scalable web applications. It is a modular and open-source successor to ASP.NET.
- Entity Framework: An Object-Relational Mapping (ORM) framework that simplifies database interactions in .NET applications, allowing developers to work with databases using object-oriented code.
- Xamarin: A framework for building cross-platform mobile applications using C# and the .NET platform. Xamarin allows developers to share a significant portion of code across iOS, Android, and Windows platforms.
- Blazor: A framework for building interactive web applications using C# and .NET instead of JavaScript. Blazor enables the development of single-page applications (SPAs) with code running directly in the browser.
- Azure Functions: A serverless compute service that allows developers to run event-triggered functions without provisioning or managing servers. It is suitable for building scalable, event-driven applications in the cloud.
- Universal Windows Platform (UWP): A framework for creating Windows apps that can run on various Windows 10 devices, such as PCs, tablets, Xbox, HoloLens, and IoT devices.
- ML.NET: A framework for building machine learning models and incorporating them into .NET applications, providing tools for training and consuming models within the application.
.NET Development Service That We Are Offerings
Welcome to Startbit IT Solutions .NET development services, we bring your ideas to life through a range of offerings tailored to meet your unique needs. Our team of experienced developers is committed to delivering high-quality solutions that leverage the power of .NET technology. Let’s dive into our key service offerings:
- Custom .NET Software Development: Our team specializes in creating tailored software solutions using the .NET framework. We analyze your business requirements to design and develop applications that precisely fit your needs, ensuring optimal performance and user satisfaction.
- .NET Desktop Application Development: Whether you need a standalone application or a comprehensive enterprise-level solution, our .NET desktop application development focuses on delivering software with a user-friendly interface, high performance, and scalability.
- ERP Development Solutions: Enterprise Resource Planning (ERP) is crucial for efficient business operations. Our ERP development solutions aim to streamline your processes, providing a unified platform for managing various aspects of your business, from finance to inventory and beyond.
- IoT Apps: As technology evolves, our Internet of Things (IoT) app development services help you harness the power of connected devices. We create applications that enable seamless communication and data exchange between devices, paving the way for smarter business operations.
- Databases: Data is at the core of any application. Our database development services focus on creating secure, efficient, and scalable databases, ensuring that your applications can manage and retrieve data seamlessly.
- Embedded Systems: Incorporating hardware and software seamlessly, our embedded systems development enables the creation of innovative solutions across industries. From smart devices to industrial applications, we ensure a cohesive integration of technology.
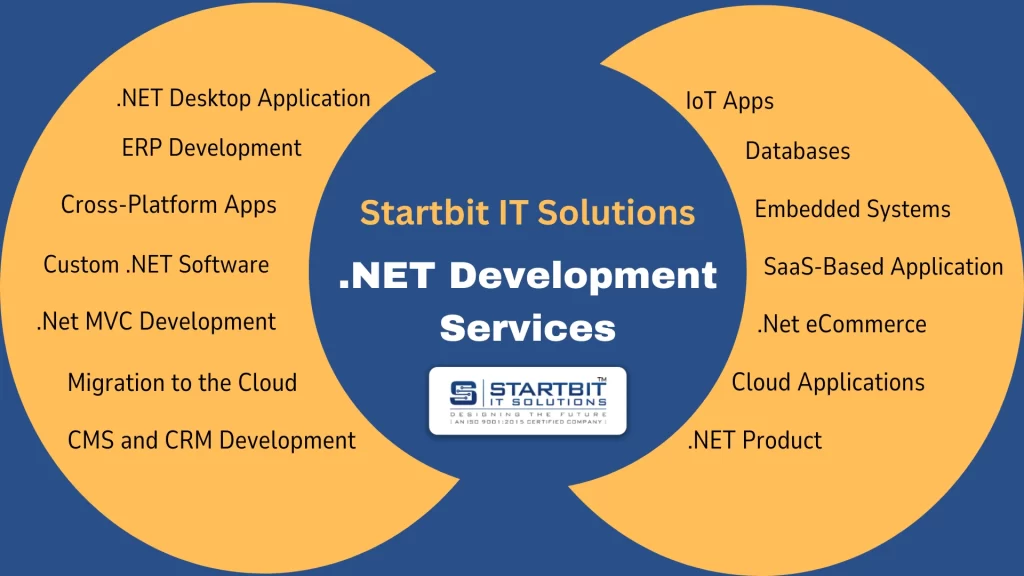
- SaaS-Based Application Development: Software as a Service (SaaS) provides accessibility and scalability. Our SaaS-based application development services focus on creating cloud-native solutions that offer flexibility, cost-effectiveness, and easy maintenance.
- .Net eCommerce Development: Elevate your online presence with our .NET eCommerce development services. We build robust and scalable platforms that enhance the shopping experience for your customers, ensuring secure transactions and user-friendly interfaces.
- Cloud Applications: Utilizing the advantages of cloud computing, our cloud application development services enable your software to be scalable, secure, and accessible from anywhere. We ensure a smooth transition to the cloud, enhancing your application’s performance and reliability.
- APIs: Application Programming Interfaces (APIs) facilitate seamless integration. Our API development services focus on creating robust interfaces, allowing your software to connect effortlessly with third-party applications, and enhancing overall functionality.
- .NET Product Development: Transform your ideas into market-ready solutions with our .NET product development services. We guide you through the entire development lifecycle, from conceptualization to deployment, ensuring your product meets market demands.
- CMS and CRM Development: Content Management Systems (CMS) and Customer Relationship Management (CRM) systems are vital for business operations. Our development services in these areas ensure efficient content management and robust customer relationship tracking, enhancing overall organizational efficiency.
- Competitive Intelligence Tools and Dashboards: Gain a competitive edge with our intelligence tools and dashboards. We create customized solutions that provide real-time insights into market trends, competitor activities, and performance metrics, empowering informed decision-making.
- Migration to the Cloud: Transitioning to the cloud can be complex. Our migration services ensure a smooth and secure process, helping you move your applications to the cloud for improved scalability, flexibility, and cost-efficiency.
- .Net MVC Development: Model-View-Controller (MVC) architecture enhances the scalability and maintainability of web applications. Our .NET MVC development services focus on creating robust and modular web solutions that align with industry best practices.
- Cross-Platform Apps on Xamarin: Reach a wider audience with our Xamarin development services. We create cross-platform applications that run seamlessly on various devices, reducing development time and ensuring a consistent user experience across platforms.
Why Choose Startbit IT Solutions for .NET Development?
In the ever-evolving landscape of technology, choosing the right IT solutions provider is crucial for the success of any business. Startbit IT Solutions emerges as a standout choice, backed by its stellar reputation and numerous accolades.
Recognition and Awards
- Good Firms’ Acknowledgment: Startbit IT Solutions has been recognized as a top web development company by Good Firms. This accolade speaks volumes about the company’s commitment to delivering high-quality services and exceeding client expectations.
- Selected Firms’ Recognition: Selected Firms has endorsed Startbit as a top website development company. This recognition further solidifies Startbit’s position as a leader in the IT solutions industry.
- iTRate.co’s Endorsement: iTRate.co, a reputable source in the tech industry, acknowledges Startbit IT Solutions as a reliable and proficient company in the field of website development. This endorsement adds credibility to Startbit’s expertise.
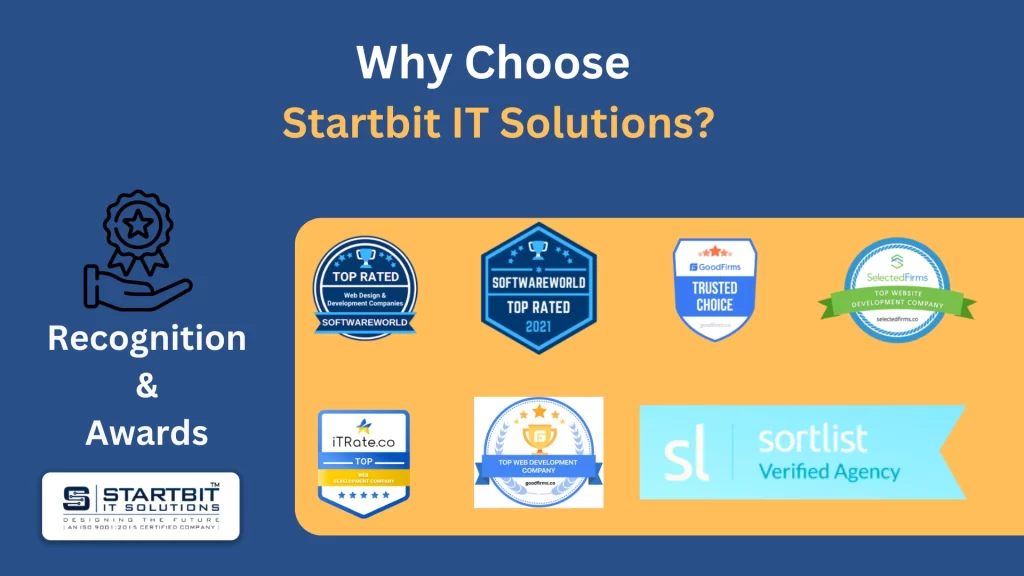
- Software World’s Top Rated 2021: Being recognized as a top-rated company by Software World in 2021 showcases Startbit’s consistency in delivering exceptional services. This accolade is a testament to the company’s dedication to excellence.
- Software World’s Acknowledgment: Startbit IT Solutions has not only excelled in web development but has also been acknowledged by Software World for its contributions to web design. This dual expertise makes Startbit a comprehensive choice for businesses looking to establish a strong online presence.
- Trusted Choice by Good Firms: Being recognized as a trusted choice by Good Firms underscores Startbit’s commitment to reliability and client satisfaction. This endorsement reflects the trust that clients place in Startbit for their web design and development needs.
Why Choose Startbit IT Solutions?
Reliability and Trust: Startbit IT Solutions has built a reputation for reliability and trust. Clients can confidently rely on Startbit to deliver solutions that meet their specific requirements, backed by a track record of successful projects.
Skilled Team of Developers: The heart of any IT solutions provider is its development team. Startbit boasts a team of skilled and experienced developers who are adept at handling complex projects and delivering results that exceed expectations.
Competitive Pricing: In addition to quality services, Startbit offers competitive pricing, making it an attractive choice for businesses of all sizes. The company understands the importance of cost-effectiveness without compromising on quality.
Tailored Solutions: Startbit recognizes that each business is unique, and one-size-fits-all solutions may not suffice. The company prides itself on delivering tailored solutions that address the specific needs and challenges of each client.
Customer-Centric Approach: Startbit IT Solutions places a strong emphasis on a customer-centric approach. The company actively involves clients throughout the development process, ensuring that the final product aligns with their vision and objectives.
Testimonials and Reviews
Positive Feedback from Clients: Numerous clients have shared positive feedback about their experience with Startbit IT Solutions. The consistent praise for the company’s professionalism, expertise, and commitment to client success speaks volumes.
Case Studies Showcasing Successful Projects
Startbit’s capabilities through case studies showcasing successful projects. These real-world examples highlight the company’s problem-solving approach and ability to deliver results.